Validating JSON object
One of the nice tools for rapid application development in Bluemix is Node-RED which escaped from IBM research. One passes a
The conversion runs non-discriminatory, so any field that is added to the form will end up in the JSON object.
In a real world application that's not a good idea, an object shouldn't have unexpected properties. I had asked before, so it wasn't too hard to derive a function I could use in Node-RED:
If you want to test the flow, simply use
The flow tests a perfect matching object, an object with a few extra properties, one with missing properties and one empty one. Import this flow:
As usual: YMMV
msg
JSON object between nodes that process (mostly) the msg.payload
property. A feature I like a lot is the ability to use a http input node that can listen to a POST
on an URL and automatically translates the posted form into a JSON object.
The conversion runs non-discriminatory, so any field that is added to the form will end up in the JSON object.
In a real world application that's not a good idea, an object shouldn't have unexpected properties. I had asked before, so it wasn't too hard to derive a function I could use in Node-RED:
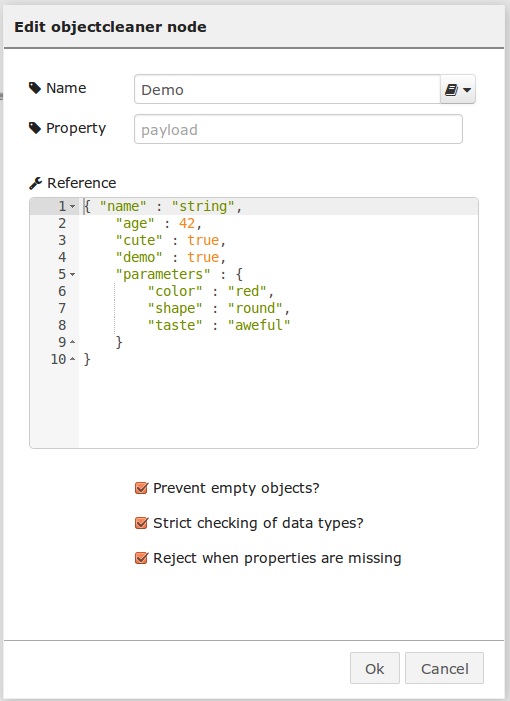
this.deepclean = function(template, candidate, hasBeenCleaned) {
var cleandit = false;
for (var prop in candidate) {
if (template.hasOwnProperty(prop)) {
// We need to check strict clean and recursion
var tProp = template[prop];
var cProp = candidate[prop];
// Case 1: strict checking and types are different
if (this.strictclean && ((typeof tProp) !== (typeof cProp))) {
delete candidate[prop];
cleandit = true;
// Case 2: both are objects - recursion needed
} else if (((typeof tProp) === "object") && ((typeof cProp) === "object")) {
cleandit = node.deepclean(tProp, cProp, (hasBeenCleaned || cleandit));
candidate[prop] = cProp;
}
// Case 3: property is not there
} else {
delete candidate[prop];
cleandit = true;
}
}
return (hasBeenCleaned || cleandit);
}
The function is called with the template object and the incoming object and the initial parameter false
. While the function could be easily used inside a function node, the better option is to wrap it into a node of its own, so it is easy to use anywhere. The details how to do that can be found on the Node-RED website. The easiest way to try the function: add your Node-RED project to version control, download the object cleaner node and unzip it into the nodes directory. Works in Bluemix and in a local Node-RED installation.
If you want to test the flow, simply use
npm install node-red-contrib-objectcleaner
or add it as dependency to your Node-RED installation. You then can test it with a flow like this:
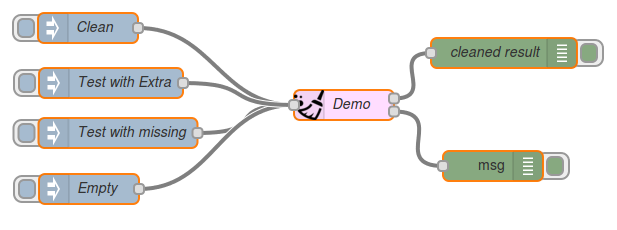
The flow tests a perfect matching object, an object with a few extra properties, one with missing properties and one empty one. Import this flow:
[
{
"id": "2729363c.e9c20a",
"type": "objectcleaner",
"name": "Demo",
"template": "{ \"name\" : \"string\",\n \"age\" : 42,\n \"cute\" : true,\n \"demo\" : true,\n \"parameters\" : {\n \"color\" : \"red\",\n \"shape\" : \"round\",\n \"taste\" : \"aweful\"\n }\n}",
"field": "",
"strictclean": true,
"notempty": true,
"musthaveallproperties": true,
"x": 359,
"y": 132,
"z": "f5e214d6.87925",
"wires": [
[
"f84af920.c4a7c"
],
[
"282d3700.460122"
]
]
},
{
"id": "bda25c33.917ac8",
"type": "inject",
"name": "Test with missing",
"topic": "",
"payload": "{ \"name\" : \"string\", \"demo\" : true, \"age\" : 42, \"cute\" : true, \"parameters\" : { \"color\" : \"red\", \"shape\" : \"round\" , \"drehzahl\" : \"hoch\", \"xxx\" : { \"a\" : \"b\", \"c\" : \"e\"} } }",
"payloadType": "string",
"repeat": "",
"crontab": "",
"once": false,
"x": 133,
"y": 160,
"z": "f5e214d6.87925",
"wires": [
[
"2729363c.e9c20a"
]
]
},
{
"id": "f84af920.c4a7c",
"type": "debug",
"name": "cleaned result",
"active": true,
"console": "false",
"complete": "payload",
"x": 519,
"y": 80,
"z": "f5e214d6.87925",
"wires": [
]
},
{
"id": "282d3700.460122",
"type": "debug",
"name": "",
"active": true,
"console": "false",
"complete": "true",
"x": 508,
"y": 193,
"z": "f5e214d6.87925",
"wires": [
]
},
{
"id": "c436845a.d5fe1",
"type": "inject",
"name": "Empty",
"topic": "",
"payload": "",
"payloadType": "none",
"repeat": "",
"crontab": "",
"once": false,
"x": 104,
"y": 216,
"z": "f5e214d6.87925",
"wires": [
[
"2729363c.e9c20a"
]
]
},
{
"id": "bf11b968.dffb08",
"type": "inject",
"name": "Clean",
"topic": "",
"payload": "{ \"name\" : \"string\", \"age\" : 42, \"cute\" : true, \"demo\" : true, \"parameters\" : { \"color\" : \"red\", \"shape\" : \"round\", \"taste\" : \"aweful\" } }",
"payloadType": "string",
"repeat": "",
"crontab": "",
"once": false,
"x": 103,
"y": 55,
"z": "f5e214d6.87925",
"wires": [
[
"2729363c.e9c20a"
]
]
},
{
"id": "5bcdb6a5.de79c",
"type": "inject",
"name": "Test with Extra",
"topic": "",
"payload": "{ \"name\" : \"string\", \"age\" : 42, \"cute\" : true, \"demo\" : true, \"parameters\" : { \"color\" : \"red\", \"shape\" : \"round\", \"taste\" : \"aweful\", \"extra\" : { \"a\" : \"b\", \"c\" : \"d\", \"e\" : true} } }",
"payloadType": "string",
"repeat": "",
"crontab": "",
"once": false,
"x": 126,
"y": 110,
"z": "f5e214d6.87925",
"wires": [
[
"2729363c.e9c20a"
]
]
}
]
As usual: YMMV
Posted by Stephan H Wissel on 11 July 2015 | Comments (0) | categories: Bluemix NodeRED